The Well-Architected FastAPI: Building Production-Ready APIs with MongoDB
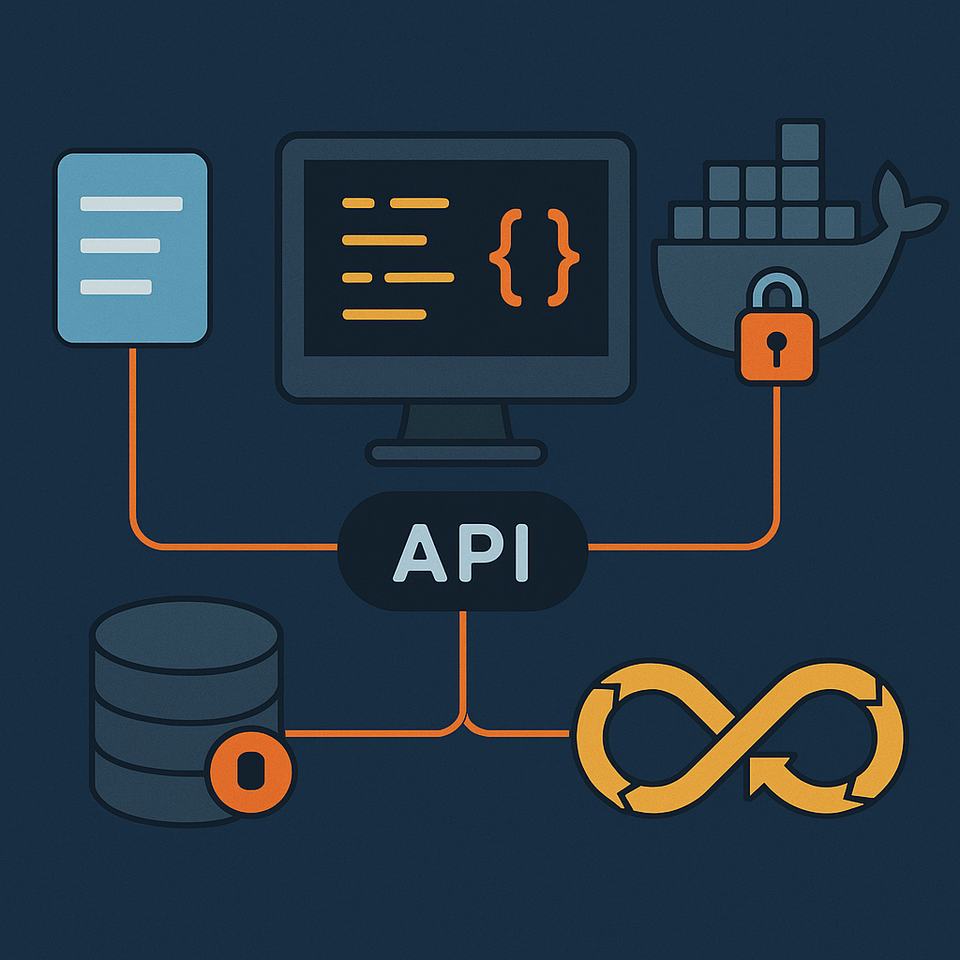
Exploring best practices and architectural patterns for robust API development
Why Architecture Matters in API Development
When a tech lead requests "a simple user management API," it's easy to underestimate what differentiates between code that works in a demo and code that thrives in production. Many tutorials show you how to create endpoints, but few address the architecture that makes an API reliable, maintainable, and scalable.
This article explores the architecture of a production-ready FastAPI application with MongoDB, examining the patterns and practices that create a solid foundation. Far from being just another CRUD example, this project demonstrates how thoughtful design can make even standard user management interesting.
Beyond Basic CRUD: Features That Matter
At its functional core, this application provides standard user operations:
- Creating user accounts
- Retrieving user information
- Updating user details
- Managing user lists
- Deleting user accounts
What sets it apart are the architectural features rarely covered in tutorials:
- Proper connection pooling with async MongoDB drivers
- Request tracing with correlation IDs for debugging distributed systems
- Middleware implementation for cross-cutting concerns
- Docker deployment with security best practices
- AsyncIO patterns that leverage FastAPI's strengths
Let's examine the architecture decisions that turn basic functionality into production-grade software.
Database Architecture: Efficient Connection Management
The Singleton Connection Pattern
Efficient database connection management is crucial for production applications. Consider this common anti-pattern:
# DON'T DO THIS
async def get_user(user_id):
client = AsyncIOMotorClient(settings.MONGODB_URI) # New connection each time
db = client[settings.MONGODB_DB]
user = await db.users.find_one({"_id": ObjectId(user_id)})
# Client connection never properly managed
return user
This creates a new connection for every request, causing connection pool exhaustion under load.
Instead, this application implements a singleton pattern:
class MongoConnector:
_client: Optional[AsyncIOMotorClient] = None
@classmethod
def get_client(cls) -> AsyncIOMotorClient:
if cls._client is None:
cls._client = AsyncIOMotorClient(
settings.MONGODB_URI, io_loop=get_event_loop()
)
return cls._client
This approach maintains a single connection pool throughout the application lifecycle. The Motor driver handles connection pooling internally, but only if you provide a consistent client instance.
Fully Asynchronous Database Operations
MongoDB operations are I/O-bound, making them ideal for async processing:
@router.get("/users", response_model=List[User])
async def list_users():
collection = get_user_collection()
users = await collection.find().to_list(100)
# Convert ObjectId to str for each user
for user in users:
user['_id'] = str(user['_id'])
return [User(**user) for user in users]
This approach doesn't block the event loop, allowing FastAPI to handle more concurrent requests. The performance difference becomes significant under load, as the server can process thousands of requests efficiently with minimal resource usage.
API Architecture: Separation of Concerns
Modular Router Pattern
The application uses FastAPI's router pattern to organize endpoints into logical groups:
# In app/main.py
app.include_router(user_router)
# In app/routers/users.py
router = APIRouter()
@router.post("/users", response_model=User)
async def create_user(user_create: UserCreate):
# Implementation here
This structure keeps the code modular and maintainable, making it easy to add new resources as the application grows. Each router handles a specific domain, with clear boundaries between different areas of functionality.
Data Validation with Pydantic Models
Data validation is handled through Pydantic models:
class UserBase(BaseModel):
name: str = Field(..., example="John Doe")
email: EmailStr = Field(..., example="john.doe@example.com")
class UserCreate(UserBase):
password: str = Field(..., min_length=8)
class User(UserBase):
id: str = Field(..., alias="_id")
This approach provides several benefits:
- Automatic validation of incoming data
- Clear documentation of data structures
- Seamless integration with FastAPI's OpenAPI documentation
- Type safety throughout the application
- Automatic data transformation between MongoDB and API representations
Observability: Request Tracing for Distributed Systems
Correlation IDs: Connecting the Dots
In a distributed system, tracing requests across services is essential for debugging. This application implements correlation IDs through middleware:
class CorrelationIdMiddleware(BaseHTTPMiddleware):
async def dispatch(self, request: Request, call_next):
request_id = str(uuid.uuid4())
_request_id_ctx_var.set(request_id)
response = await call_next(request)
response.headers["X-Request-ID"] = request_id
return response
The correlation ID is then available throughout the request lifecycle and appears in logs:
2023-05-10 14:23:45 - app - INFO - 123e4567-e89b-12d3-a456-426614174000 - User created with id 6455f3afc94d6a1c3b9e2d7a
This seemingly simple addition transforms debugging from a frustrating search to a straightforward trace, especially as the system grows in complexity.
Custom Logging
The application implements custom logging to include correlation IDs in log messages:
class CustomFormatter(logging.Formatter):
def format(self, record):
if not hasattr(record, 'correlation_id'):
record.correlation_id = 'N/A'
return super().format(record)
This ensures consistent log formatting across the application, making it easier to analyze logs and troubleshoot issues.
Container Architecture: Security and Efficiency
Multi-Stage Docker Builds
The application uses a multi-stage Docker build for efficiency and security:
# Stage 1: Build dependencies
FROM python:3.12-slim as builder
WORKDIR /app
RUN apt-get update && apt-get install -y --no-install-recommends gcc
COPY requirements.txt .
RUN pip wheel --no-cache-dir --no-deps --wheel-dir /app/wheels -r requirements.txt
# Stage 2: Final image
FROM python:3.12-slim
WORKDIR /app
# Create a non-root user
RUN groupadd -r appuser && useradd -r -g appuser appuser
COPY --from=builder /app/wheels /wheels
COPY --from=builder /app/requirements.txt .
RUN pip install --no-cache /wheels/*
COPY . .
USER appuser
CMD ["uvicorn", "app.main:app", "--host", "0.0.0.0", "--port", "8000"]
This approach provides several benefits:
- Smaller final image size
- Separation of build and runtime environments
- Non-root execution for improved security
- Reduced attack surface
Docker Compose for Local Development
The application uses Docker Compose to orchestrate the API and its MongoDB dependency:
version: '3.8'
services:
app:
build: .
ports:
- "8000:8000"
depends_on:
- mongo
environment:
- MONGODB_URI=mongodb://mongo:27017
command: ["uvicorn", "app.main:app", "--host", "0.0.0.0", "--port", "8000"]
mongo:
image: mongo:5.0
ports:
- "27017:27017"
volumes:
- ./mongo-data:/data/db
This makes local development and testing straightforward, ensuring consistency between environments.
Error Handling: Robustness Through Structure
Consistent error handling creates a more reliable API. Each endpoint follows a pattern:
@router.get("/users/{user_id}", response_model=User)
async def get_user(user_id: str):
collection = get_user_collection()
user = await collection.find_one({"_id": ObjectId(user_id)})
if user:
user['_id'] = str(user['_id'])
return User(**user)
raise HTTPException(status_code=404, detail="User not found")
This approach ensures:
- HTTP status codes match the nature of the error
- Error messages are consistent across endpoints
- Exceptions don't leak internal details to clients
- The API behavior is predictable even when things go wrong
Quality Assurance: Automated Testing
The application includes unit tests using FastAPI's TestClient:
def test_create_user(self):
response = self.client.post(
"/users",
json={
"name": "Test User",
"email": "test@example.com",
"password": "password123"
}
)
self.assertEqual(response.status_code, 200)
data = response.json()
self.assertIn("id", data)
These tests run automatically on each push via GitHub Actions:
name: CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build-and-test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Python
uses: actions/setup-python@v4
with:
python-version: '3.12'
- name: Install dependencies
run: |
pip install -r requirements.txt
- name: Run tests
run: |
python -m unittest discover -s tests
This CI pipeline ensures that changes don't break existing functionality, maintaining the application's reliability as it evolves.
Configuration Management: Environment Over Hardcoding
The application uses Pydantic for settings management:
class Settings(BaseSettings):
MONGODB_URI: str = "mongodb://mongo:27017"
MONGODB_DB: str = "testdb"
class Config:
env_file = ".env"
This provides:
- Default values for development
- Environment variable overrides for production
- .env file support for local configuration
- Type validation for settings
This approach is more maintainable than hardcoded values and avoids exposing sensitive information in the codebase.
Hands-on Exploration
To explore this API architecture firsthand:
- Access the API:
- Swagger UI: http://localhost:8000/docs
- Experiment with the Code:
- Add a new field to the User model
- Create a new endpoint for searching users
- Implement pagination for the list endpoint
Create a user via the UI or curl:
curl -X POST http://localhost:8000/users \ -H "Content-Type: application/json" \ -d '{"name":"John Doe","email":"john@example.com","password":"securepassword"}'
Run with Docker Compose:
docker compose up
Clone the Repository:
git clone https://github.com/yourusername/fastapi-user-crud.git
cd fastapi-user-crud
Key Architectural Lessons
This project demonstrates several important principles:
1. Connection Management is Critical
The way database connections are handled can make or break an application's performance under load. A well-implemented connection pool improves both reliability and efficiency.
2. Async Requires Thoughtful Implementation
Asynchronous code is only effective when implemented correctly. Simply adding async/await
keywords aren't enough; understanding the event loop and avoiding blocking operations is essential.
3. Consistent Error Handling Creates Reliability
A predictable error-handling strategy makes an API more user-friendly and easier to maintain. Consistent status codes and error messages improve the developer experience for API consumers.
4. Security Should Be Built In, Not Added On
Security considerations like non-root Docker execution, proper password handling, and appropriate CORS settings should be part of the initial design, not afterthoughts.
5. Tests Provide a Safety Net for Evolution
Automated tests don't just verify that code works today; they ensure it continues to work as the application evolves, catching regressions before they reach production.
Future Architecture Expansion
This foundation can support several advanced features:
- Authentication with JWT and refresh tokens
- Role-based access control
- Rate limiting middleware
- Caching layer with Redis
- More comprehensive test coverage
- API versioning
- Health check endpoints with dependency status
Each of these would build upon the existing architecture, extending rather than replacing the core patterns.
Conclusion: Structure Creates Value
While a basic CRUD API might seem straightforward, the architectural decisions behind it determine its true value in production. By implementing thoughtful connection management, proper async patterns, request tracing, error handling, and testing, even a simple user management API can become a robust, maintainable service.
The practices demonstrated in this project apply to FastAPI applications of all types, from microservices to monoliths, and serve as a foundation for more complex systems. The code is available on GitHub as a reference implementation and starting point for your projects.
Want to discuss FastAPI architecture or share your own best practices? The comments section is open for discussion. This example is intentionally over-engineered for educational purposes - real-world applications should balance complexity against actual requirements.
Comments ()